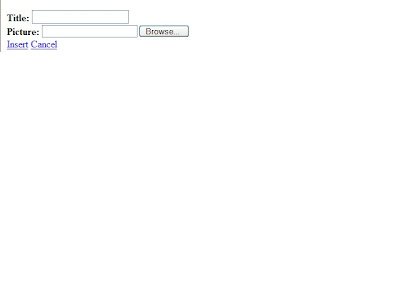
Drag TextBox,FileUpload and LinkButton Control to save the Image
Paste the following Code on to the Insert Button Click
protected void btnInsert_Click(object sender, EventArgs e)
{
if (UploadedFile.PostedFile == null || UploadedFile.PostedFile.FileName == "" || UploadedFile.PostedFile.InputStream == null)
{
Response.Write("please select the file by clicking browse button");
}
Stream imgStream = UploadedFile.PostedFile.InputStream;
int imgLen = UploadedFile.PostedFile.ContentLength;
string imgContentType = UploadedFile.PostedFile.ContentType;
string imgName = PictureTitle.Text;
byte[] imgBinaryData = new byte[imgLen];
int n = imgStream.Read(imgBinaryData, 0, imgLen);
DateTime imgDate = Convert.ToDateTime(System.DateTime.Now.ToShortDateString());
int RowsAffected = SaveToDB(imgName, imgBinaryData, imgContentType,imgDate);
if (RowsAffected > 0)
{
Response.Write("
The Image " + imgName + " was saved");
The Image " + imgName + " was saved");
}
else
{
Response.Write("
An error occurred uploading the image");
An error occurred uploading the image");
}
}
Then the write the method to Save the Image into the Database
private int SaveToDB(string Title, byte[] ImageData, string MIMEType,DateTime imgTime)
{
SqlConnection conn = new SqlConnection("server=sheeban\\sqlexpress;integrated security=SSPI;database=Test");
string strSql = "INSERT INTO Image (Title, MIMEType, ImageData, DataUploaded) VALUES (@Title, @MIMEType, @ImageData, @imgTime)";
SqlCommand cmd=new SqlCommand(strSql,conn);
SqlParameter param0 = new SqlParameter("@Title", Title);
cmd.Parameters.Add(param0);
SqlParameter param1 = new SqlParameter("@ImageData", ImageData);
cmd.Parameters.Add(param1);
SqlParameter param2 = new SqlParameter("@MIMEType", MIMEType);
cmd.Parameters.Add(param2);
SqlParameter param3 = new SqlParameter("@imgTime", imgTime);
cmd.Parameters.Add(param3);
conn.Open();
int numRowsAffected = cmd.ExecuteNonQuery();
conn.Close();
return numRowsAffected;
}
Drag Textbox,Button Control on the Page to display the Image
Then write the method of ShowPictures
public void ShowPictures(int PictureID)
{
SqlConnection conn = new SqlConnection("server=sheeban\\sqlexpress;integrated security=SSPI;database=Test");
string strSql = "SELECT [MIMEType], [ImageData], [DataUploaded], [Title] FROM [Image] WHERE [ImageID] = @ImageID";
SqlCommand cmd = new SqlCommand(strSql, conn);
SqlParameter param = new SqlParameter("ImageID", PictureID);
cmd.Parameters.Add(param);
conn.Open();
SqlDataReader myReader = cmd.ExecuteReader();
if (myReader.Read())
{
Response.ContentType = myReader["MIMEType"].ToString();
Response.BinaryWrite((byte[])myReader["ImageData"]);
}
myReader.Close();
conn.Close();
}
Then call this Method on to the button Click
protected void btnPictureID_Click(object sender, EventArgs e)
{
ShowPictures(Convert.ToInt32(tbxPictureID.Text));
}
No comments:
Post a Comment