Monday, December 7, 2009
Stored Procedure using Dataset
CREATE PROCEDURE SelProduct
AS
BEGIN
-- SET NOCOUNT ON added to prevent extra result sets from
-- interfering with SELECT statements.
SET NOCOUNT ON;
SELECT Product_id, Product_Name, Product_Prise, Product_Quantity FROM Product
END
GO
//Call the Select Product Stored Procedure into the code
try
{
SqlCommand cmd = new SqlCommand();
cmd.CommandType = CommandType.StoredProcedure;
SqlConnection conn = new SqlConnection(ConfigurationManager.ConnectionStrings["SampleConnString"].ConnectionString);
SqlDataAdapter da = new SqlDataAdapter("SelProduct", conn);
DataSet ds = new DataSet();
da.Fill(ds);
gvProductDisplay.DataSource = ds;
gvProductDisplay.DataBind();
}
catch (Exception ex)
{
Response.Write(ex.Message);
}
Tuesday, November 24, 2009
Apply Parameter Field using Crystal Report
<CR:CrystalReportViewer ID="CrystalReportViewer1" runat="server" AutoDataBind="true" />
Code Behind of the Page
ReportDocument objReportDocument;
Page Load
protected void Page_Load(object sender, EventArgs e)
{
if (Request.QueryString["EmployeeID"] != null)
{
string reportPath = Server.MapPath("ProgramaticallyReport.rpt");
objReportDocument = new ReportDocument();
ParameterDiscreteValue objDiscreteValueEmployeeID = new ParameterDiscreteValue();
string objCustomerID = (Convert.ToString(Request.QueryString["EmployeeID"]));
objDiscreteValueEmployeeID.Value = objCustomerID;
//ParameterDiscreteValue objDiscreteValueLastMonth = new ParameterDiscreteValue();
//DateTime objDate2 = (System.Convert.ToDateTime(Request.QueryString["Date2"]));
//objDiscreteValueLastMonth.Value = objDate2;
ParameterField objParameterField = new ParameterField();
objParameterField.Name = "EmpID";
objParameterField.CurrentValues.Add(objDiscreteValueEmployeeID);
//objParameterField.CurrentValues.Add(objDiscreteValueLastMonth);
ParameterFields objParameterFields = new ParameterFields();
objParameterFields.Add(objParameterField);
objReportDocument.Load(reportPath);
objReportDocument.SetDataSource(GetCustomer());
CrystalReportViewer1.ParameterFieldInfo = objParameterFields;
CrystalReportViewer1.ReportSource = objReportDocument;
}
else
{
Exception objException = new Exception();
lblMessage.Text = objException.Message;
}
GetCustomer Dataset Method
public DataSet GetCustomer()
{
string objCustomerID = (Convert.ToString(Request.QueryString["EmployeeID"]));
SqlParameter objParameter = new SqlParameter();
SqlCommand objCommand = new SqlCommand();
SqlConnection conn = new SqlConnection(ConfigurationManager.ConnectionStrings["NorthwindConnectionString"].ConnectionString);
SqlDataAdapter da = new SqlDataAdapter("SELECT EmployeeID,FirstName, LastName, City, Country " +
"FROM Employees where EmployeeID=@EmployeeID", conn);
da.SelectCommand.Parameters.Add(new SqlParameter("@EmployeeID", objCustomerID));
da.SelectCommand.Parameters["@EmployeeID"].Value = objCustomerID;
DataSet ds = new DataSet();
da.Fill(ds, "Employees");
return ds;
}
Using HyperLink in Gridview
<asp:GridView ID="gvEmployee" runat="server" AutoGenerateColumns="False">
<Columns>
<asp:BoundField DataField="EmployeeID" HeaderText="Employee ID" />
<asp:BoundField DataField="FirstName" HeaderText="First Name" />
<asp:BoundField DataField="LastName" HeaderText="Last Name" />
<asp:BoundField DataField="BirthDate" HeaderText="BirthDate" />
<asp:BoundField DataField="City" HeaderText="City" />
<asp:BoundField DataField="Country" HeaderText="Country" />
<asp:TemplateField>
<ItemTemplate>
<asp:HyperLink ID="HyperLink1" runat="server" NavigateUrl='<%#Eval("EmployeeID","Default.aspx?EmployeeID={0}") %>' Text='<%# Bind("EmployeeID") %>'></asp:HyperLink>
</ItemTemplate>
</asp:TemplateField>
</Columns>
</asp:GridView>
Code Behing of Page
SqlParameter objParameter = new SqlParameter();
SqlCommand objCommand = new SqlCommand();
SqlConnection conn = new SqlConnection(ConfigurationManager.ConnectionStrings["NorthwindConnectionString"].ConnectionString);
SqlDataAdapter da = new SqlDataAdapter("SELECT EmployeeID, FirstName, LastName, BirthDate, City, Country FROM Employees", conn);
//da.SelectCommand.Parameters.Add(new SqlParameter("@CustomerID", customerid));
//da.SelectCommand.Parameters["@CustomerID"].Value = customerid;
DataSet ds = new DataSet();
da.Fill(ds, "Employees");
gvEmployee.DataSource = ds;
gvEmployee.DataBind();
Tuesday, November 17, 2009
Custom Membership and Role Provider
<membership defaultProvider="CustomMembershipProvider">
<providers>
<add name="CustomMembershipProvider" type="System.Web.Security.SqlMembershipProvider" connectionStringName="MusharkaCertConnString" applicationName="MusharkaCertificate" enablePasswordRetrieval="false" enablePasswordReset="true" requiresQuestionAndAnswer="false" requiresUniqueEmail="false" passwordFormat="Clear" minRequiredPasswordLength="6" minRequiredNonalphanumericCharacters="0" passwordStrengthRegularExpression="(\w+)*"/>
<providers>
<membership>
Role Provider
<roleManager enabled="true" defaultProvider="CustomProvider">
<providers>
<add connectionStringName="MusharkaCertConnString" name="CustomProvider" type="System.Web.Security.SqlRoleProvider"/>
</providers>
</roleManager>
Grouping Data into the ListView Control
Add New Item "Linq 2 Sql Classes" and Drag 2 table from Server Explorer
1) Customers
2) Orders
Design View of HTML Page
<asp:ListView ID="lvCustomerOrders" runat="server" ItemPlaceholderID="itemPlaceHolder">
<LayoutTemplate>
<h2>Customers</h2>
<ul>
<asp:PlaceHolder ID="itemPlaceHolder" runat="server">
</asp:PlaceHolder>
</ul>
</LayoutTemplate>
<ItemTemplate>
<li>
<b> <%# Eval("FirstName") %> <%# Eval("LastName") %> </b>
<asp:ListView ID="lvOrders" DataSource ='<%# Eval("Orders") %>' runat="server" ItemPlaceholderID="productPlaceHolder">
<LayoutTemplate>
<table>
<tr>
<th>
Order Name
</th>
<th>
Quantity
</th>
</tr>
<asp:PlaceHolder ID="productPlaceHolder" runat="server"></asp:PlaceHolder>
</table>
</LayoutTemplate>
<ItemTemplate>
<table width="120px">
<tr>
<td>
<%# Eval("OrderName") %>
</td>
<td>
<%# Eval("Quantity") %>
</td>
</tr>
</table>
</ItemTemplate>
<EmptyDataTemplate>
<li>
<table><tr><td style="color:Red">There are no Orders</td></tr></table>
</li>
</EmptyDataTemplate>
</asp:ListView>
</li>
</ItemTemplate>
</asp:ListView>
Code Behind of HTML Page
protected void Page_Load(object sender, EventArgs e)
{
if (!Page.IsPostBack)
{
BindData();
}
}
private void BindData()
{
using (var db = new NwdDataClassesDataContext())
{
lvCustomerOrders.DataSource = from c in db.Customers
select c;
lvCustomerOrders.DataBind();
}
}
Insert Data into Multiple Tables
INSERT INTO Customers
(FirstName, LastName)
VALUES ('Sheeban', 'Ahmed')
SET @ID = SCOPE_IDENTITY()
INSERT INTO Orders
(CustomerID, OrderName, Quantity)
VALUES (@ID,'IPhone',100)
Wednesday, August 26, 2009
Saving Word Document to the Sql Server
CREATE TABLE [dbo].[File1](
[id] [int] IDENTITY(1,1) NOT NULL,
[Filename] [varchar](50) COLLATE SQL_Latin1_General_CP1_CI_AS NOT NULL,
[FileBytes] [varbinary](max) NOT NULL
) ON [PRIMARY]
The Design view of a Web Form
<asp:Label id="lblFile" Text="wordDocument" AssociatedControlID="upFile" runat="server" />
<asp:FileUpload id="upFile" runat="Server" />
<asp:Button id="btnAdd" Text="Add Documents" runat="server" OnClick="btnAdd_Click" />
<hr />
<asp:Repeater id="rptFiles" DataSourceID="srcFiles" runat="Server">
<HeaderTemplate>
<ul class="fileList">
</HeaderTemplate>
<ItemTemplate>
<li>
<asp:HyperLink id="lnkFile" Text='<%#Eval("FileName")%>' NavigateUrl='<%#Eval("id","~/FileHandler.ashx?id={0}")%>' runat="Server" />
</li>
</ItemTemplate>
<FooterTemplate>
</ul>
</FooterTemplate>
</asp:Repeater>
<asp:SqlDataSource id="srcFiles"
ConnectionString="<%$ ConnectionStrings:FileDBConnectionString %>"
SelectCommand="SELECT [id], [Filename] FROM [File1]"
InsertCommand="INSERT INTO [File1] (Filename, FileBytes) values (@fileName,@FileBytes)"
runat="server">
<InsertParameters>
<asp:ControlParameter Name="FileName" ControlID="upFile" PropertyName="FileName" />
<asp:ControlParameter Name="FileBytes" ControlID="upFile" PropertyName="FileBytes" />
</InsertParameters>
</asp:SqlDataSource>
The Code Behind of Button Add Documents
if (upFile.HasFile)
{
if (CheckFileType(upFile.FileName))
srcFiles.Insert();
}
The Function CheckFileType
bool CheckFileType(string fileName)
{
return Path.GetExtension(fileName).ToLower() == ".doc";
}
Then Add Custom Handler on to the Page
public void ProcessRequest(HttpContext context)
{
context.Response.ContentType = "application/msword";
SqlConnection con = new SqlConnection(@"Data Source=SHEEBAN\SQLEXPRESS;Initial Catalog=FileDB;Integrated Security=True");
SqlCommand cmd = new SqlCommand("select filebytes from [file1] where id=@id", con);
cmd.Parameters.AddWithValue("@id", context.Request["id"]);
using (con)
{
con.Open();
byte[] file = (byte[])cmd.ExecuteScalar();
context.Response.BinaryWrite(file);
}
}
public bool IsReusable
{
get
{
return false;
}
}
Monday, August 24, 2009
Saving Data into XML Format
Xml Xml1 = new Xml();
protected void Page_Load(object sender, EventArgs e)
{
//Create a connection string.
string sqlConnect=@"Data Source=SHEEBAN\SQLEXPRESS;Initial Catalog=Northwind;Integrated Security=True";
SqlConnection sqlconnect = new SqlConnection(ConfigurationManager.ConnectionStrings["NorthwindConnection"].ConnectionString);
//Create a connection object to connect to the web shoppe database
try
{
SqlConnection nwconnect = new SqlConnection(sqlConnect);
String scommand = "select top 10 * from customers";
//Create an adapter to load the dataset
SqlDataAdapter da = new SqlDataAdapter(scommand, nwconnect);
//Fill the dataset
da.Fill(ds, "Customers");
XmlDataDocument doc = new XmlDataDocument(ds);
//Xml1.Document = doc;
doc.Save(MapPath("Customers.xml"));//This is where we are saving the data in an XML file Customers.xml
Label1.Text = "Your Data saved succesfully";
}
catch
{
//if there is any error then Label1 will give the Error
Label1.Text = "Error while connecting to database";
}
}
SQL Injection Example
<table>
<tr>
<td>
UserName:
</td>
<td>
<asp:TextBox ID="tbxUserName" runat="server"></asp:TextBox>
</td>
</tr>
<tr>
<td>
Password:
</td>
<td>
<asp:TextBox ID="tbxPassword" runat="server" TextMode="Password"></asp:TextBox>
</td>
</tr>
<tr>
<td colspan="2" align="right">
<asp:Button ID="btnLogin" runat="server" Text="Login" Width="150px" OnClick="btnLogin_Click"/>
</td>
</tr>
<tr>
<td colspan="2" align="left">
<asp:Literal ID="Literal1" runat="server" Text=""></asp:Literal>
</td>
</tr>
</table>
//Now the Code Behind of the Button Login
string conn = ConfigurationManager.ConnectionStrings["FileDBConnectionString"].ConnectionString;
string query = "Select Count(*) From Users Where Username = '" + tbxUserName.Text + "' And Password = '" + tbxPassword.Text + "'";
int result = 0;
SqlConnection connection = new SqlConnection(conn);
connection.Open();
SqlCommand cmd = new SqlCommand(query, connection);
result = (int)cmd.ExecuteScalar();
if (result > 0)
{
connection.Close();
Response.Redirect("LoggedIn.aspx");
}
else
{
Literal1.Text = "Invalid credentials";
connection.Close();
}
If we copy the "'or'1'='1"(without quotation marks) both in the password textbox and username textbox it will redirect you on to the LoggedIn page this is what we called SQL Injection
In order to control the SQL Injection we have to add few code of line Just before the Query
string username = tbxUserName.Text.Replace("'", "''");
string password = tbxPassword.Text.Replace("'", "''");
And query would be change into
string query = "Select Count(*) From Users Where Username = '" + username + "' And Password = '" + password + "'";
Saturday, August 22, 2009
Toggle HTML Element
Enter the Text:<input id="Text1" type="text" />
<input id="Button1" type="button" value="button" onclick="toggle()" />
//JavaScript to toggle the HTML Element
<script type="text/javascript">
function toggle(){
document.getElementById('Text1').style.display == '' ? document.getElementById('Text1').style.display = 'none'
: document.getElementById('Text1').style.display = '';
}
</script>
Concatenation in Sql Query
--if i run this query i will get result like that.

--now i want to concatenate all the same category data type so i have to write query like that
SELECT A.Category,
(SELECT B.DataType + ',' FROM DataTypeCategory B WHERE B.Category=A.Category
FOR XML PATH('')) 'Concatinated' FROM DataTypeCategory A GROUP BY A.Category

SQL_Query_Substring
--I declare @SampleString cause i can easily increase the length of variable '@SampleString'
SELECT @SampleString = 'Sheeban Ahmed'
SELECT SUBSTRING(@SampleString,1,1) AS S
--S
SELECT SUBSTRING(@SampleString,1,2) AS SH
--Sh
SELECT SUBSTRING(@SampleString,1,0) AS BLANK
--<BLANK>
SELECT SUBSTRING(@SampleString,0,1) AS BLANK
--<BLANK>
SELECT SUBSTRING(@SampleString,-1,4) AS SH
--S
SELECT SUBSTRING(@SampleString,-1,0) AS BLANK
--<BLANK>
SELECT SUBSTRING(@SampleString,-10,12) AS S
--S
SELECT SUBSTRING(@SampleString,-10,25) AS [SHEEBAN AHMED]
--Sheeban Ahmed
Thursday, August 20, 2009
Reading and Writing File
Write the value in the TextBox
<asp:TextBox ID="tbxWrite" runat="server"></asp:TextBox>
<asp:Button ID="btnWrite" runat="server" Text="Write"
OnClick="btnWrite_Click" />
<br />
Read all that value are written in the Textbox
<asp:Button ID="btnRead" runat="server" Text="Read" OnClick="btnRead_Click" />
<asp:Literal ID="litText" runat="server"></asp:Literal>
The Code Behind of that Page
string contents = "";
string fileNameWithPath=@"c:\sample.txt";//where ur text file is present
protected void Page_Load(object sender, EventArgs e)
{
}
protected void btnWrite_Click(object sender, EventArgs e)
{
//This will be used to write the file
using (StreamWriter writer = File.AppendText(fileNameWithPath))
{
//whatever u write in that textbox it will write on to that text file
writer.Write(tbxWrite.Text);
}
}
protected void btnRead_Click(object sender, EventArgs e)
{
// Read data from text file
using (StreamReader reader = File.OpenText(fileNameWithPath))
{
// reads a single line of contents
contents = reader.ReadLine();
// reads complete contents of the the file
contents += reader.ReadToEnd();
}
litText.Text = contents;
}
Wednesday, July 29, 2009
Abstract Class vs Interface Table
Feature | Interface | Abstract Class |
---|---|---|
Multiple inheritance | A class may inherit severalinterfaces | A class may inherit only one abstract class |
Default implementation | An interface cannot provide any code, just the signature. | An abstract class can provide complete, default code and/or just the details that have to be overridden. |
Constants | Only Static final constants | Both instance and static constants are possible |
Speed | Requires more time to find the actual method in the corresponding classes. | Fast |
Adding functionality | If we add a new method to an Interface then we have to track down all the implementations of the interface and define implementation for the new method | If we add a new method to an abstract class then we have the option of providing default implementation and therefore all the existing code might work properly. |
Homogeneity | If the various implementations only share method signatures then it is better to use Interface | If the various implementations abstract class then we have the common behavior or status then abstract class is better to use. |
Loading Data by Generic List using stored procedure with DataTable and DataReader
cmd.ExecuteReader() method to get the SqlDataReader and read through all the records in the reader and add the Person object into the List.
The second method is slightly different and use DataTable instead of DataReader to fetch data from database, loop through all the rows of the DataTable and add Person objects into the list.
You may wonder why these two methods when both are doing the same thing. I prefer using DataReader when I have lesser amount of data from the database and loop through because DataReader expect live connection when it loops through all the records. For large amount of data, it may be a bottleneck for the database to keep the connection alive and loop through. So for large amount of data I prefer to fill the DataTable, close the database connection and then loop through all the records do the required operation.
public List
{
var list = new List
using (SqlConnection conn = new SqlConnection(connStr))
{
using (SqlCommand cmd = new SqlCommand("LoadAll", conn))
{
cmd.CommandType = CommandType.StoredProcedure;
conn.Open();
using (SqlDataReader reader = cmd.ExecuteReader())
{
while (reader.Read())
{
Person p = new Person()
{
Age = reader.GetInt32(reader.GetOrdinal("Age")),
FirstName = reader.GetString(reader.GetOrdinal("FirstName")),
LastName = reader.GetString(reader.GetOrdinal("LastName"))
};
list.Add(p);
}
}
conn.Close();
}
return list;
}
}
public List
{
var list = new List
using (SqlConnection conn = new SqlConnection(connStr))
{
using (SqlCommand cmd = new SqlCommand("LoadAll", conn))
{
cmd.CommandType = CommandType.StoredProcedure;
using (SqlDataAdapter ad = new SqlDataAdapter(cmd))
{
DataTable table = new DataTable();
conn.Open();
// open the connection
ad.Fill(table);
conn.Close();
// close the connection
// loop through all rows
foreach (DataRow row in table.Rows)
{
Person p = new Person()
{
Age = int.Parse(row["Age"].ToString()),
FirstName = row["FirstName"].ToString(),
LastName = row["LastName"].ToString()
};
list.Add(p);
}
}
}
return list;
}
}
Wednesday, July 15, 2009
Javascript Split method
<form id="Form1" runat="server">
<asp:TextBox ID="tbxabc" runat="server" />
<br />
<asp:Label ID="lblFirstName" runat="server" Text="">
<br />
<asp:Label ID="lblSecondName" runat="server" Text="">
<input type="button" id="Calculate" value="Split" onclick="split()" />
</form>
<script type="text/javascript">
function split() {
var i;
var abc = document.getElementById('tbxabc').value;
for (i = 0; i < abc.length; i++) {
alert(abc[i]);
}
}
</script>
</body>
//For example i enter my name in the Textbox for example sheeban
//In the pop up each and every charachter will pop up
Monday, July 13, 2009
Get the value of Selected Row From Gridview
string textBoxCompanyName, textBoxContactName, textBoxCustomerID;
//Then on the button click event get the values from the grid
//Here dvCustomerDetail is the name of a Gridview
foreach (GridViewRow row in dvCustomerDetail.Rows)
{
textBoxCustomerID = ((TextBox)row.FindControl("tbxCustomerID")).Text;
textBoxCompanyName = ((TextBox)row.FindControl("tbxCompanyName")).Text;
textBoxContactName = ((TextBox)row.FindControl("tbxContactName")).Text;
}
Thursday, June 18, 2009
Javascript Document Method
document.anchors — Contains all <a> elements with a name attribute in the document.
document.applets — Contains all <applet> elements in the document. This collection is deprecated, because the <applet> element is no longer recommended for use.
document.forms — Contains all <form> elements in the document. The same as document.getElementsByTagName("form").
document.images — Contains all <img> elements in the document. The same as document.getElementsByTagName("img").
document.links — Contains all <a> elements with an href attribute in the document.
Tuesday, June 16, 2009
Determine Browser by using JS
var leftPos = (typeof window.screenLeft == "number")?window.screenLeft :window.screenX;
var topPos = (typeof window.screenTop == "number") ? window.screenTop : window.screenY;
This example uses the ternary operator to determine if the screenLeft and screenTop properties exist. If they do (which is the case in IE, Safari, Opera, and Chrome), they are used. If they don’t exist (as in Firefox), screenX and screenY are used.
Wednesday, June 10, 2009
Using DataGrid_ItemCreated
<asp:DataGrid ID="DataGrid1" runat="server" AutoGenerateColumns="false" onitemcreated="DataGrid1_ItemCreated">
<Columns>
<asp:BoundColumn DataField="CustomerID" HeaderText="Customer ID"/>
<asp:BoundColumn DataField="CustomerName" HeaderText="Customer Name"/>
<asp:BoundColumn DataField="CustomerPhone" HeaderText="Customer Phone"/>
<asp:BoundColumn DataField="CustomerAddress" HeaderText="Customer Address"/>
<asp:BoundColumn DataField="CustomerAge" HeaderText="Customer Age"/>
</Columns>
</asp:DataGrid>
//Then Add the Event DataGrid_ItemCreated
protected void DataGrid1_ItemCreated(object sender, DataGridItemEventArgs e)
{
if(e.Item.ItemType == ListItemType.Header)
{
e.Item.Cells.RemoveAt(2);
e.Item.Cells[1].ColumnSpan = 2;
e.Item.Cells[1].Text = "<table border=\"1px\" style=\"width:100%; text-align:center; \"" +
" <tr align=\"centre\">" +
" <td colspan=\"2\">" +
" Name" +
" </td>" +
" </tr>" +
" </table>" +
"<table border=\" 1px\" " +
" <tr align=\"centre\">" +
" <td>" +
" First Name" +
" </td>" +
" <td>" +
" Last Name" +
" </td>" +
" </tr>" +
" </table>";
}
}
Thursday, May 28, 2009
Restrict User to Copying Text
<body oncontextmenu="return false" oncopy="return false;" onpaste="return false;" oncut="return false;"/>
Friday, May 22, 2009
Javascript Timer Control
<script type="text/javascript" language="javascript"/>
var hour=11;
var minutes=59;
var seconds=59;
function timedCount()
{
//Textbox name is txt and time would be started in the textbox
document.getElementById('txt').value=hour+":"+minutes+":"+seconds;
if(hour==11 && minutes==58 && seconds==59)
{
//as soon as the time reach 11:58:59 it will transfer itself to another page Default.aspx
window.location.href='Default.aspx';
}
else if(seconds==0)
{
minutes-=1;
seconds=60;
}
if(minutes==01)
{
hour-=1;
minutes=59;
seconds=60;
}
seconds-=1;
//timedcount function decrase by one second which is mentioned here in Millisecond
t=setTimeout("timedCount()",1000);
}
</script>
Thursday, May 21, 2009
Transferring Data From Excel Sheet to Access
{
//Calling Cotacts.mdb(Access Database) from the App_Data Folder
string Access = Server.MapPath("App_Data/contact.mdb");
//Calling Book.xls(Excel Sheet) from the Root Folder
string Excel = Server.MapPath("Book.xls");
//Connection string For Excel Sheet
string connect = "Provider=Microsoft.Jet.OLEDB.4.0;Data Source=" + Excel + ";Extended Properties=Excel 8.0;";
using (OleDbConnection conn = new OleDbConnection(connect))
{
using (OleDbCommand cmd = new OleDbCommand())
{
try
{
cmd.Connection = conn;
//Inserting Data into the Access Database fromthe Excel Sheet where Excel Sheet Name is Sheet1
cmd.CommandText = "INSERT INTO [MS Access;Database=" + Access + "].[Person] SELECT * FROM [Sheet1$]";
conn.Open();
cmd.ExecuteNonQuery();
}
catch (Exception ex)
{
Response.Write("Your error is " + ex.Message);
}
}
}
}
Gmail setting using Asp.Net
1)From(For Name)
2)To (Recepient Email Address)
3)Subject
4)Body*/
protected void btnMail_Click(object sender, EventArgs e)
{
SmtpClient mailClient = new SmtpClient("smtp.gmail.com", 587);
mailClient.EnableSsl = true;
//In Network Credential give ur UserName and Password
NetworkCredential cred = new NetworkCredential("sheeban101pk@gmail.com", "xxxxxx");
mailClient.Credentials = cred;
try
{
mailClient.Send(tbxFrom.Text.Trim(), tbxTo.Text.Trim(), tbxSubject.Text.Trim(), tbxBody.Text.Trim());
Response.Write("Your Email has been sent sucessfully -Thank You");
}
catch (Exception exc)
{
Response.Write("Send failure: " + exc.ToString());
}
}
Total value of Gridview in Footer
float total1 = 0,total2=0;
//On page load Bind the Employee Salary Function with DataGrid
protected void Page_Load(object sender, EventArgs e)
{
DataGrid1.DataSource = GetEmployeeSalary();
DataGrid1.DataBind();
}
//Getting the data from Database
public DataSet GetEmployeeSalary()
{
SqlConnection conn = new SqlConnection(ConfigurationManager.ConnectionStrings["Connection"].ConnectionString.ToString());
string sqlcmd = "SELECT Name, Bonus, SUM(Salary) as Salary"+
" FROM CustomerSalary"+
" group by Name, Bonus";
SqlCommand cmd = new SqlCommand(sqlcmd, conn);
SqlDataAdapter da = new SqlDataAdapter(cmd);
DataSet ds = new DataSet();
da.Fill(ds);
return ds;
}
//Using Item Data Bound to get the total value in footer of Gridview
protected void DataGrid1_ItemDataBound(object sender, DataGridItemEventArgs e)
{
if (e.Item.ItemType!=ListItemType.Header && e.Item.ItemType!=ListItemType.Footer)
{
total1 += float.Parse(e.Item.Cells[1].Text);
total2 += float.Parse(e.Item.Cells[2].Text);
e.Item.ForeColor = System.Drawing.Color.Blue;
}
else if (e.Item.ItemType == ListItemType.Footer)
{
e.Item.Cells[0].Text = "Total";
e.Item.Cells[1].Text = total1.ToString();
e.Item.Cells[2].Text = total2.ToString();
//e.Item.Cells[3].Text = total.ToString();
}
}
Tuesday, April 28, 2009
JQuery Basics
What does jQuery have to offer? First and foremost, jQuery has given me power over the Document Object Model (DOM)! jQuery Selectors are the greatest thing since sliced bread, no lie. Being able to EASILY find an object or group of objects in the DOM by ID, CSS Class, or by HTML Tag is something web developers have long needed.
To select an object from the DOM by ID would look like this:
$('#ID_Goes_Here')
To select an object from the DOM by CSS Class would look like this:
$('.CSS_Class_Name_Goes_Here')
To select an object from the DOM by HTML Tag would look like this:
$('Tag_Goes_Here')
For example, if I have a label that I want to be rendered but not visible I could create the label.
asp:label id="Label4" runat="server" cssclass="myLabel" text="This is " And later on in jQuery I can hide it like this.
$('#Label4').css('display', 'none');
It’s nice to be able to easily select an object and modify it, but what if you have a whole group of items you want to modify? With jQuery, you can EASILY loop through a collection of objects.
In this example I have a group of labels.
asp:label id="Label1" runat="server" cssclass="myLabel" text="This is "
asp:label id="Label2" runat="server" cssclass="myLabel" text="This is "
asp:label id="Label3" runat="server" cssclass="myLabel" text="This is "
asp:label id="Label4" runat="server" cssclass="myLabel" text="This is "
Now I want to update the text of each label to include its ID. I am going to loop through each object in the DOM with a CSS Class of .myLabel.
$('.myLabel').each(function() { $(this).append(this.id); });
What the jQuery code above does is execute a function that will append the object’s ID to its text value. Notice the Selector inside the function $(this). The syntax is used to find the loop’s current object in the DOM.
Now I have shown you some snippets here and there, let me show you what my page actually looks like.
script type="text/javascript"
function pageLoad() {
$('.myLabel').each(function() { $(this).append(this.id); });
$('.myLabel').css('color', '#FFFFFF').css('font-size', '30px');
$('#Label4').css('display', 'none');
$("#Panel1").html("(ul)(li)Item One(/li)(li)Item 2(/li)(/ul)");
}
script
asp:label id="Label1" runat="server" cssclass="myLabel" text="This is "
asp:label id="Label2" runat="server" cssclass="myLabel" text="This is "
asp:label id="Label3" runat="server" cssclass="myLabel" text="This is "
asp:label id="Label4" runat="server" cssclass="myLabel" text="This is "
asp:panel id="Panel1" runat="server"
Wednesday, April 15, 2009
Why we Use Triggers
Triggers can check data against values of data in the rest of the table or in other tables. This is useful when you cannot use RI constraints or check constraints because of references to data from other rows from this or other tables.
Triggers can use user-defined functions to activate non-database operations. This is useful, for example, for issuing alerts or updating information outside the database.
Some Examples:
INSERT INTO Customers
VALUES (Sheeban,Ahmed,Memon,Karachi,
Sindh,110016,01126853138)
INSERT INTO Customers
VALUES(Faizan,Ahmed,Khanani,
Chicago,illions,326541,9412658745)
INSERT INTO Products
VALUES (ASP.Net Microsoft Press,550)
INSERT INTO Products
VALUES (ASP.Net Wrox Publication,435)
INSERT INTO Products
VALUES (ASP.Net Unleased,320)
INSERT INTO Products
VALUES (ASP.Net aPress,450)
CREATE TRIGGER invUpdate ON [Orders]
FOR INSERT
AS
UPDATE p SET p.instock=[p.instock,i.qty]
FROM products p JOIN inserted I ON p.prodid = i.prodid
CREATE TRIGGER SindhDel ON [Customers]
FOR DELETE
AS
IF (SELECT state FROM deleted) = Sindh
BEGIN
PRINT Can not remove customers from Sindh
PRINT Transaction has been canceled
ROLLBACK
END
CREATE TRIGGER CheckStock ON [Products]
FOR UPDATE
AS
IF (SELECT InStock FROM inserted) < 0
BEGIN
PRINT Cannot oversell Products
PRINT Transaction has been cancelled
ROLLBACK
END
Tuesday, March 24, 2009
Get the Value of a Grid Cell
{
string CustomerId = e.Item.Cells[0].Text;
}
Thursday, March 19, 2009
Code Behind of RDLC Reporting
Wednesday, March 18, 2009
Update Query with where Clause in a seperate Class
public bool Update(string customerId, string customerName, string customerPhone, string customerAddress, string customerAge)
{
SqlCommand objCommand = new SqlCommand();
SqlConnection conn = new SqlConnection(ConfigurationManager.ConnectionStrings["test"].ConnectionString);
SqlParameter objParameter = new SqlParameter();
try
{
string strSql = "UPDATE Customer "+
"SET CustomerName = @CustomerName, CustomerPhone = @CustomerPhone, CustomerAddress = @CustomerAddress, "+
"CustomerAge = @CustomerAge WHERE CustomerID = @CustomerID";
objCommand.CommandText = strSql;
objCommand.Connection = conn;
objParameter = new SqlParameter("@CustomerID", customerId);
objCommand.Parameters.Add(objParameter);
objParameter = new SqlParameter("@CustomerName", customerName);
objCommand.Parameters.Add(objParameter);
objParameter = new SqlParameter("@CustomerPhone", customerPhone);
objCommand.Parameters.Add(objParameter);
objParameter = new SqlParameter("@CustomerAddress", customerAddress);
objCommand.Parameters.Add(objParameter);
objParameter = new SqlParameter("@CustomerAge", customerAge);
objCommand.Parameters.Add(objParameter);
objCommand.Connection.Open();
return (objCommand.ExecuteNonQuery() > 0 ? true : false);
}
catch (Exception ex)
{
conn.Close();
return false;
}
finally
{
conn.Close();
}
}
Create a object of Update on the update button click event which get CustomerId through Query String
int customerid = Convert.ToInt32(Request.QueryString["CustomerId"].ToString());
Customer objCustomer = new Customer();
objCustomer.Update(customerid.ToString(), tbxCustomerName.Text.Trim(), tbxCustomerPhone.Text.Trim(), tbxCustomerAddress.Text.Trim(), tbxCustomerAge.Text.Trim());
Dataset with where Clause
On A button Click event we get all the Values of Customer Table(CustomerName, CustomerAddress, CustomerAge, CustomerPhone) through Customer ID which is present in the Query String.
protected void btnGet_Click(object sender, EventArgs e)
{
int customerid = Convert.ToInt32(Request.QueryString["CustomerId"].ToString());
SqlParameter objParameter = new SqlParameter();
SqlCommand objCommand = new SqlCommand();
SqlConnection conn = new SqlConnection(ConfigurationManager.ConnectionStrings["test"].ConnectionString);
SqlDataAdapter da = new SqlDataAdapter("SELECT CustomerID, CustomerName, CustomerPhone, CustomerAddress, CustomerAge " +
"FROM Customer where CustomerID=@CustomerID", conn);
da.SelectCommand.Parameters.Add(new SqlParameter("@CustomerID", customerid));
da.SelectCommand.Parameters["@CustomerID"].Value = customerid;
DataSet ds = new DataSet();
da.Fill(ds, "Customer");
foreach (DataRow pRow in ds.Tables["Customer"].Rows)
{
tbxCustomerName.Text = pRow["CustomerName"].ToString();
tbxCustomerPhone.Text = pRow["CustomerPhone"].ToString();
tbxCustomerAddress.Text = pRow["CustomerAddress"].ToString();
tbxCustomerAge.Text = pRow["CustomerAge"].ToString();
}
}
Monday, March 9, 2009
Linq with Classes using Join in Query
HTTP Modules Events
Every HTTP Module must implement following two methods of IHTTPModule interface:
Init: To register/initialize event handler to the events of HTTP Module for HTTP based application.
Dispose: To perform a clean up code means resource releasing, object removing from memory and such other resources releasing which used explicitly.
Following are list of events with their brief description:
BeginRequest: Event fired whenever any asp.net based request sent to web server. If you need to do perform at the beginning of a request for example, modify show banners, log HTTP Header information, Get/Set cultures, Response.Filter to generate response for browser according to your need.
AuthenticateRequest: If you want to check authentication of request that request comes from authenticated user or not means wants to implement custom authentication scheme. For example, look up a requested user credentials against a database to validate.
AuthorizeRequest: This method is used specifically to implement authorization mechanisms means authenticated user/request has what privileges/rights/access in that specific application for example, either user has access on all pages or not of that website or has write to create file or not or visit report pages and like this.
ResolveRequestCache: This event determines if a page served from the Output cache. If you want to write your own caching module (for example, build a file-based cache rather than a memory cache), synchronize this event to determine whether to serve the page from the cache.
AcquireRequestState: Session state is retrieved from the state store. If you want to build your own state management module, synchronize this event to grab the Session state from your state store.
PreRequestHandlerExecute: This event occurs before the HTTP handler is executed.
PostRequestHandlerExecute: This event occurs after the HTTP handler is executed.
ReleaseRequestState: Session state is stored back in the state store. If you are building a custom session state module, you must store your state back in your state store.
UpdateRequestCache: This event writes from output back to the Output cache. If you are building a custom cache module, you have to write the output back to your cache.
Error: this event always occurs when any exception (unhandled error occurs in application, this event specifically uses to handle or log error messages of that web application. (Heavily used in Error Logging Modules and Handlers (ELMAH) kind of applications). You can learn about ELMAH more from following link in detail:http://dotnetslackers.com/articles/aspnet/ErrorLoggingModulesAndHandlers.aspx
EndRequest: Request has been completed. You may want to build a debugging module that gathers information throughout the request and then writes the information on the page.
By above events list you must be getting wonder about difference between Global.asax as somehow events of Global.asax are pretty same, so let me tell you difference between Global.asax and HTTP Module (another common question asked in interviews)
But you need to register/initialize these Events explicitly in “Init” method; following is sample code of IHTTPModule implementation for couple of events.
Wednesday, February 25, 2009
Comapring Two Objects
private int Compare (int x, int y)
{
return x < y ? x : y;
}
When we comapre two Object value we usually performed boxing and unboxing which create overhead on the Application. We used a collection similarly when value is added to a collection boxing take places when value is retrieved unboxing take places. Collection makes a job easy for boxing and unboxing
private T Compare
{
if (x.CompareTo (y) < 0)
return x;
else
return y;
}
Friday, January 30, 2009
C | Local currency format. |
D | Decimal format. Converts an integer to base 10, and pads with leading zeros if a precision specifier is given |
E | Scientific (exponential) format. The precision specifier sets the number of decimal places (6 by default). The case of the format string (e or E) determines the case of the exponential symbol |
F | F Fixed-point format; the precision specifier controls the number of decimal places. Zero is acceptable |
G | General format. Uses E or F formatting, depending on which is the most compact. |
N | Number format. Formats the number with commas as thousands separators, for example 32,767.44. |
P | Percent format. |
X | Hexadecimal format. The precision specifier can be used to pad with leading zeros. |
Wednesday, January 28, 2009
Round of Method
Example of getting value in Bytes
Pass Multiple Values from a GridView to Another Page using ASP.NET
SelectCommand="SELECT [CustomerID], [CompanyName], [ContactName], [Address], [City] FROM [Customers]">
An entry will be added to the web.config file as shown below:
connectionStrings
add name="NorthwindConnectionString" connectionString="Data Source=(local);Initial Catalog=Northwind;Integrated Security=True"providerName="System.Data.SqlClient"
Step 2: Now add a GridView control to the page and using the smart tag, select the DataSource to be SqlDataSource1 in the GridView tasks panel. Using the same panel, click on the Enable Paging and Enable Sorting checkboxes. The source will look similar to the following. Observe that the DataKeyNames is set to ‘CustomerId’, that is the primary key of the Customers table.
asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="False" DataKeyNames="CustomerID" DataSourceID="SqlDataSource1" AllowPaging="True" AllowSorting="True"
Columns
asp:BoundField DataField="CustomerID" HeaderText="CustomerID" ReadOnly="True" SortExpression="CustomerID"
asp:BoundField DataField="CompanyName" HeaderText="CompanyName" SortExpression="CompanyName"
asp:BoundField DataField="ContactName" HeaderText="ContactName" SortExpression="ContactName"
asp:BoundField DataField="Address" HeaderText="Address" SortExpression="Address"
asp:BoundField DataField="City" HeaderText="City" SortExpression="City"
Columns
asp:GridView
Step 3: We will now add another page in our project. In the Solution Explorer, right click the project > Add New Item > Web Form > Rename it to ‘CustomerDetails.aspx’.
Step 4: Go back to Default.aspx and add two hyperlink fields. We will see how to pass a single value as well as multiple values using the two hyperlink fields.
Single Value:
Add the following hyperlink control after the
Columns
asp:HyperLinkField DataNavigateUrlFields="CustomerID" DataNavigateUrlFormatString="CustomerDetails.aspx?CID={0}" Text="Pass Single Value"
Multiple Values:
Just below the first hyperlink field, add another hyperlink field as shown below:
asp:HyperLinkField DataNavigateUrlFields="CustomerID, CompanyName, ContactName, Address, City" DataNavigateUrlFormatString="CustomerDetails.aspx?CID={0}&CName={1}&ContactName={2}&Addr={3}&City={4}" Text="Pass Multiple Values"
In the source code shown above, we are using the hyperlink field and setting some properties that will make it extremely easy to pass values to a different page. The 'DataNavigateUrlFields' sets the names of the fields, that is to be used to construct the URL in the HyperLinkField. In the first hyperlink, since we are passing only a single value, the DataNavigateUrlFields contains only CustomerID. However in the second hyperlink, since there are multiple values to be passed, DataNavigateUrlFields contains all the names of the fields that are to be passed as query string to CustomerDetails.aspx
Similarly, the 'DataNavigateUrlFormatString' sets the string that specifies the format in which the URL is to be created. The 'Text' property represents the text that will be displayed to the user. The entire source code will look similar to the following:
SelectCommand="SELECT [CustomerID], [CompanyName], [ContactName], [Address], [City] FROM [Customers]"
asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="False" DataKeyNames="CustomerID" DataSourceID="SqlDataSource1" AllowPaging="True" AllowSorting="True"
Columns
asp:HyperLinkField DataNavigateUrlFields="CustomerID"
DataNavigateUrlFormatString="CustomerDetails.aspx?CID={0}"
Text="Pass Single Value"
asp:HyperLinkField DataNavigateUrlFields="CustomerID, CompanyName, ContactName, Address, City"
DataNavigateUrlFormatString="CustomerDetails.aspx?CID={0}&CName={1}&ContactName={2}&Addr={3}&City={4}"
Text="Pass Multiple Values"
asp:BoundField DataField="CustomerID" HeaderText="CustomerID" ReadOnly="True" SortExpression="CustomerID"
asp:BoundField DataField="CompanyName" HeaderText="CompanyName" SortExpression="CompanyName"
asp:BoundField DataField="ContactName" HeaderText="ContactName" SortExpression="ContactName"
asp:BoundField DataField="Address" HeaderText="Address" SortExpression="Address"
asp:BoundField DataField="City" HeaderText="City" SortExpression="City"
Columns
asp:GridView
Step 5: The last step is to retrieve the query string variables from the URL in the CustomerDetails.aspx page. Add the following code for that:
C#
protected void Page_Load(object sender, EventArgs e)
{
string cid = Request.QueryString["CID"];
string cname = Request.QueryString["CName"];
string contactName = Request.QueryString["ContactName"];
string address = Request.QueryString["Addr"];
string city = Request.QueryString["City"];
}
VB.NET
Protected Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs)
Dim cid As String = Request.QueryString("CID")
Dim cname As String = Request.QueryString("CName")
Dim contactName As String = Request.QueryString("ContactName")
Dim address As String = Request.QueryString("Addr")
Dim city As String = Request.QueryString("City")
End Sub
Set a breakpoint at the Page_Load method of the CustomerDetails.aspx. Run the application and click on either the ‘Pass Single Value’ or ‘Pass Multiple Values’ hyperlink to pass values to the CustomerDetails.aspx page. Using the breakpoint, observe the values of the selected row being passed to the CustomerDetails page.
Tuesday, January 27, 2009
- MembershipCreateStatus.DuplicateEmail
- MembershipCreateStatus.DuplicateProviderUserKey
- MembershipCreateStatus.DuplicateUserName
- MembershipCreateStatus.InvalidAnswer
- MembershipCreateStatus.InvalidEmail
- MembershipCreateStatus.InvalidPassword
- MembershipCreateStatus.InvalidProviderUserKey
- MembershipCreateStatus.InvalidQuestion
- MembershipCreateStatus.InvalidUserName
- MembershipCreateStatus.ProviderError
- MembershipCreateStatus.Success
- MembershipCreateStatus.UserRejected
Friday, January 23, 2009
Linq to XML with Join Query
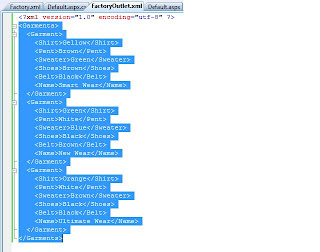
var query = from m in XElement.Load(MapPath("FactoryOutlet.xml")).Elements("Garment")
join g in XElement.Load(MapPath("Factory.xml")).Elements("Factory")
on (string)m.Element("Name") equals (string)g.Element("Name")
select new
{
Shirt=(string)m.Element("Shirt"),
Pent=(string)m.Element("Pent"),
Sweater=(string)m.Element("Sweater"),
Shoes=(string)m.Element("Shoes"),
Belt=(string)m.Element("Belt"),
Name=(string)m.Element("Name")
};
gvFactoryOutlet.DataSource = query;
gvFactoryOutlet.DataBind();
Thursday, January 22, 2009
Image Saved in a DB in Binary Format
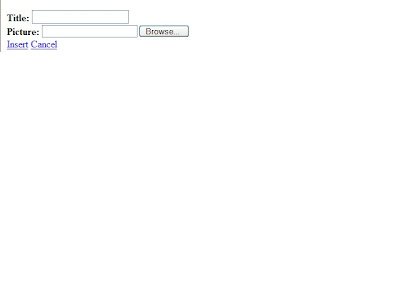
The Image " + imgName + " was saved");
An error occurred uploading the image");
Wednesday, January 21, 2009
Calendar Day Render Event in Asp.Net
Then select the Day Render event of Calendar and paste the following code into it.
protected void Calendar1_DayRender(object sender, DayRenderEventArgs e)
{
e.Cell.VerticalAlign = VerticalAlign.Top;
if (e.Day.Date == Convert.ToDateTime("01/27/2009"))
{
e.Cell.Controls.Add(new LiteralControl("
Sheeban Ahmed Birthday!
"));e.Cell.BorderColor = System.Drawing.Color.Black;
e.Cell.BorderWidth = 1;
e.Cell.BorderStyle = BorderStyle.Solid;
e.Cell.BackColor = System.Drawing.Color.LightGray;
}
}
After running that Page you will have a screenshot like that
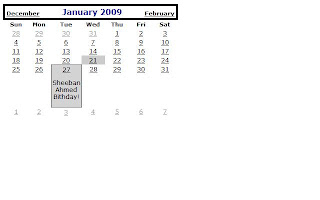
Use CrossPagePostBack Property to Transform Parameter From one Page to Another
Drag three Control on to the Source.aspx Page
TextBox, Calendar and Button
Then Paste the following code on to the button click Event
protected void Button1_Click(object sender, EventArgs e)
{
Server.Transfer("Default2.aspx");
}
Then Drag the Label Control on to the Destination.aspx Page
Then Paste the following code on to the Page_Load Event
protected void Page_Load(object sender, EventArgs e)
{
if (PreviousPage != null)
{
TextBox pp_TextBox1;
Calendar pp_Calendar1;
pp_TextBox1 = (TextBox)PreviousPage.FindControl("TextBox1");
pp_Calendar1 = (Calendar)PreviousPage.FindControl("Calendar1");
Label1.Text = "Hello " + pp_TextBox1.Text + "
" + "Date Selected " + pp_Calendar1.SelectedDate.ToShortDateString();
}
else
Label1.Text = "Sorry";
}
Then run the following code write something in a Textbox and select the date from the Calendar then press the button you will see that all of these data would be transfered on to the Next Page
Monday, January 19, 2009
Simple Example of Lambda Expression
C# 3.0 Language Enhancements
C# 3.0 Language Enhancements
The C# 3.0 language enhancements build on C# 2.0 to increase developer productivity: they make written code more concise and make working with data as easy as working with objects. These features provide the foundation for the LINQ project, a general purpose declarative query facility that can be applied to in-memory collections and data stored in external sources such as XML files and relational databases.
The C# 3.0 language enhancements consist of:
Auto-implemented properties, which automate the process of creating properties with trivial implementations.
Implicitly typed local variables, which permit the type of local variables to be inferred from the expressions used to initialize them.
Implicitly typed arrays, a form of array creation and initialization that infers the element type of the array from an array initializer.
Extension methods, which make it possible to extend existing types and constructed types with additional methods.
Lambda expressions, an evolution of anonymous methods that concisely improves type inference and conversions to both delegate types and expression trees.
Expression trees, which permit lambda expressions to be represented as data (expression trees) instead of as code (delegates).
Object and collection initializers, which you can use to conveniently specify values for one or more fields or properties for a newly created object, combining creation and initialization into a single step.
Query expressions, which provide a language-integrated syntax for queries that is similar to relational and hierarchical query languages such as SQL and XQuery.
Anonymous types, which are tuple types automatically inferred and created from object initializers.