C | Local currency format. |
D | Decimal format. Converts an integer to base 10, and pads with leading zeros if a precision specifier is given |
E | Scientific (exponential) format. The precision specifier sets the number of decimal places (6 by default). The case of the format string (e or E) determines the case of the exponential symbol |
F | F Fixed-point format; the precision specifier controls the number of decimal places. Zero is acceptable |
G | General format. Uses E or F formatting, depending on which is the most compact. |
N | Number format. Formats the number with commas as thousands separators, for example 32,767.44. |
P | Percent format. |
X | Hexadecimal format. The precision specifier can be used to pad with leading zeros. |
Friday, January 30, 2009
Wednesday, January 28, 2009
Round of Method
Example of getting value in Bytes
Pass Multiple Values from a GridView to Another Page using ASP.NET
SelectCommand="SELECT [CustomerID], [CompanyName], [ContactName], [Address], [City] FROM [Customers]">
An entry will be added to the web.config file as shown below:
connectionStrings
add name="NorthwindConnectionString" connectionString="Data Source=(local);Initial Catalog=Northwind;Integrated Security=True"providerName="System.Data.SqlClient"
Step 2: Now add a GridView control to the page and using the smart tag, select the DataSource to be SqlDataSource1 in the GridView tasks panel. Using the same panel, click on the Enable Paging and Enable Sorting checkboxes. The source will look similar to the following. Observe that the DataKeyNames is set to ‘CustomerId’, that is the primary key of the Customers table.
asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="False" DataKeyNames="CustomerID" DataSourceID="SqlDataSource1" AllowPaging="True" AllowSorting="True"
Columns
asp:BoundField DataField="CustomerID" HeaderText="CustomerID" ReadOnly="True" SortExpression="CustomerID"
asp:BoundField DataField="CompanyName" HeaderText="CompanyName" SortExpression="CompanyName"
asp:BoundField DataField="ContactName" HeaderText="ContactName" SortExpression="ContactName"
asp:BoundField DataField="Address" HeaderText="Address" SortExpression="Address"
asp:BoundField DataField="City" HeaderText="City" SortExpression="City"
Columns
asp:GridView
Step 3: We will now add another page in our project. In the Solution Explorer, right click the project > Add New Item > Web Form > Rename it to ‘CustomerDetails.aspx’.
Step 4: Go back to Default.aspx and add two hyperlink fields. We will see how to pass a single value as well as multiple values using the two hyperlink fields.
Single Value:
Add the following hyperlink control after the
Columns
asp:HyperLinkField DataNavigateUrlFields="CustomerID" DataNavigateUrlFormatString="CustomerDetails.aspx?CID={0}" Text="Pass Single Value"
Multiple Values:
Just below the first hyperlink field, add another hyperlink field as shown below:
asp:HyperLinkField DataNavigateUrlFields="CustomerID, CompanyName, ContactName, Address, City" DataNavigateUrlFormatString="CustomerDetails.aspx?CID={0}&CName={1}&ContactName={2}&Addr={3}&City={4}" Text="Pass Multiple Values"
In the source code shown above, we are using the hyperlink field and setting some properties that will make it extremely easy to pass values to a different page. The 'DataNavigateUrlFields' sets the names of the fields, that is to be used to construct the URL in the HyperLinkField. In the first hyperlink, since we are passing only a single value, the DataNavigateUrlFields contains only CustomerID. However in the second hyperlink, since there are multiple values to be passed, DataNavigateUrlFields contains all the names of the fields that are to be passed as query string to CustomerDetails.aspx
Similarly, the 'DataNavigateUrlFormatString' sets the string that specifies the format in which the URL is to be created. The 'Text' property represents the text that will be displayed to the user. The entire source code will look similar to the following:
SelectCommand="SELECT [CustomerID], [CompanyName], [ContactName], [Address], [City] FROM [Customers]"
asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="False" DataKeyNames="CustomerID" DataSourceID="SqlDataSource1" AllowPaging="True" AllowSorting="True"
Columns
asp:HyperLinkField DataNavigateUrlFields="CustomerID"
DataNavigateUrlFormatString="CustomerDetails.aspx?CID={0}"
Text="Pass Single Value"
asp:HyperLinkField DataNavigateUrlFields="CustomerID, CompanyName, ContactName, Address, City"
DataNavigateUrlFormatString="CustomerDetails.aspx?CID={0}&CName={1}&ContactName={2}&Addr={3}&City={4}"
Text="Pass Multiple Values"
asp:BoundField DataField="CustomerID" HeaderText="CustomerID" ReadOnly="True" SortExpression="CustomerID"
asp:BoundField DataField="CompanyName" HeaderText="CompanyName" SortExpression="CompanyName"
asp:BoundField DataField="ContactName" HeaderText="ContactName" SortExpression="ContactName"
asp:BoundField DataField="Address" HeaderText="Address" SortExpression="Address"
asp:BoundField DataField="City" HeaderText="City" SortExpression="City"
Columns
asp:GridView
Step 5: The last step is to retrieve the query string variables from the URL in the CustomerDetails.aspx page. Add the following code for that:
C#
protected void Page_Load(object sender, EventArgs e)
{
string cid = Request.QueryString["CID"];
string cname = Request.QueryString["CName"];
string contactName = Request.QueryString["ContactName"];
string address = Request.QueryString["Addr"];
string city = Request.QueryString["City"];
}
VB.NET
Protected Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs)
Dim cid As String = Request.QueryString("CID")
Dim cname As String = Request.QueryString("CName")
Dim contactName As String = Request.QueryString("ContactName")
Dim address As String = Request.QueryString("Addr")
Dim city As String = Request.QueryString("City")
End Sub
Set a breakpoint at the Page_Load method of the CustomerDetails.aspx. Run the application and click on either the ‘Pass Single Value’ or ‘Pass Multiple Values’ hyperlink to pass values to the CustomerDetails.aspx page. Using the breakpoint, observe the values of the selected row being passed to the CustomerDetails page.
Tuesday, January 27, 2009
- MembershipCreateStatus.DuplicateEmail
- MembershipCreateStatus.DuplicateProviderUserKey
- MembershipCreateStatus.DuplicateUserName
- MembershipCreateStatus.InvalidAnswer
- MembershipCreateStatus.InvalidEmail
- MembershipCreateStatus.InvalidPassword
- MembershipCreateStatus.InvalidProviderUserKey
- MembershipCreateStatus.InvalidQuestion
- MembershipCreateStatus.InvalidUserName
- MembershipCreateStatus.ProviderError
- MembershipCreateStatus.Success
- MembershipCreateStatus.UserRejected
Friday, January 23, 2009
Linq to XML with Join Query
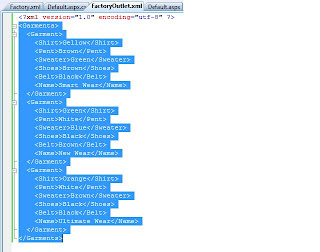
var query = from m in XElement.Load(MapPath("FactoryOutlet.xml")).Elements("Garment")
join g in XElement.Load(MapPath("Factory.xml")).Elements("Factory")
on (string)m.Element("Name") equals (string)g.Element("Name")
select new
{
Shirt=(string)m.Element("Shirt"),
Pent=(string)m.Element("Pent"),
Sweater=(string)m.Element("Sweater"),
Shoes=(string)m.Element("Shoes"),
Belt=(string)m.Element("Belt"),
Name=(string)m.Element("Name")
};
gvFactoryOutlet.DataSource = query;
gvFactoryOutlet.DataBind();
Thursday, January 22, 2009
Image Saved in a DB in Binary Format
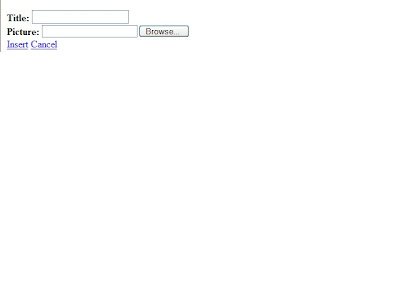
The Image " + imgName + " was saved");
An error occurred uploading the image");
Wednesday, January 21, 2009
Calendar Day Render Event in Asp.Net
Then select the Day Render event of Calendar and paste the following code into it.
protected void Calendar1_DayRender(object sender, DayRenderEventArgs e)
{
e.Cell.VerticalAlign = VerticalAlign.Top;
if (e.Day.Date == Convert.ToDateTime("01/27/2009"))
{
e.Cell.Controls.Add(new LiteralControl("
Sheeban Ahmed Birthday!
"));e.Cell.BorderColor = System.Drawing.Color.Black;
e.Cell.BorderWidth = 1;
e.Cell.BorderStyle = BorderStyle.Solid;
e.Cell.BackColor = System.Drawing.Color.LightGray;
}
}
After running that Page you will have a screenshot like that
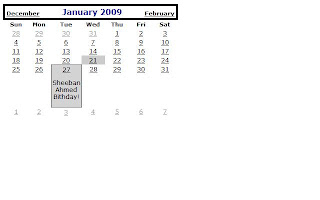
Use CrossPagePostBack Property to Transform Parameter From one Page to Another
Drag three Control on to the Source.aspx Page
TextBox, Calendar and Button
Then Paste the following code on to the button click Event
protected void Button1_Click(object sender, EventArgs e)
{
Server.Transfer("Default2.aspx");
}
Then Drag the Label Control on to the Destination.aspx Page
Then Paste the following code on to the Page_Load Event
protected void Page_Load(object sender, EventArgs e)
{
if (PreviousPage != null)
{
TextBox pp_TextBox1;
Calendar pp_Calendar1;
pp_TextBox1 = (TextBox)PreviousPage.FindControl("TextBox1");
pp_Calendar1 = (Calendar)PreviousPage.FindControl("Calendar1");
Label1.Text = "Hello " + pp_TextBox1.Text + "
" + "Date Selected " + pp_Calendar1.SelectedDate.ToShortDateString();
}
else
Label1.Text = "Sorry";
}
Then run the following code write something in a Textbox and select the date from the Calendar then press the button you will see that all of these data would be transfered on to the Next Page
Monday, January 19, 2009
Simple Example of Lambda Expression
C# 3.0 Language Enhancements
C# 3.0 Language Enhancements
The C# 3.0 language enhancements build on C# 2.0 to increase developer productivity: they make written code more concise and make working with data as easy as working with objects. These features provide the foundation for the LINQ project, a general purpose declarative query facility that can be applied to in-memory collections and data stored in external sources such as XML files and relational databases.
The C# 3.0 language enhancements consist of:
Auto-implemented properties, which automate the process of creating properties with trivial implementations.
Implicitly typed local variables, which permit the type of local variables to be inferred from the expressions used to initialize them.
Implicitly typed arrays, a form of array creation and initialization that infers the element type of the array from an array initializer.
Extension methods, which make it possible to extend existing types and constructed types with additional methods.
Lambda expressions, an evolution of anonymous methods that concisely improves type inference and conversions to both delegate types and expression trees.
Expression trees, which permit lambda expressions to be represented as data (expression trees) instead of as code (delegates).
Object and collection initializers, which you can use to conveniently specify values for one or more fields or properties for a newly created object, combining creation and initialization into a single step.
Query expressions, which provide a language-integrated syntax for queries that is similar to relational and hierarchical query languages such as SQL and XQuery.
Anonymous types, which are tuple types automatically inferred and created from object initializers.
Friday, January 16, 2009
Calling Data into the Grid through DataSet
Usage of Update command in a Seperate Class
Thursday, January 15, 2009
A Date Format Calendar of the Asp.net
lblCalendar.Text = "The date format is " + tofiletime.ToString();
lblCalendar.Text = "The date format is " + tofiletimeutc.ToString();
lblCalendar.Text = "The date format is " + localTime.ToString();
lblCalendar3.Text = "The Long Date format is " + dTime.ToLongDateString() + "
";
lblCalendar4.Text = "The Long Time format is " + dTime.ToLongTimeString() + "
";
lblCalendar5.Text = "The Short Date format is " + dTime.ToShortDateString() + "
";
lblCalendar6.Text = "The Short Time format is " + dTime.ToShortTimeString() + "
";
lblCalendar7.Text = "The ToString Time format is " + dTime.ToString() + "
";
lblCalendar7.Text = "The Universal Time format is " + dTime.ToUniversalTime() + "
";
Monday, January 12, 2009
Object Oriented Programming Question from C#
What is the difference between struct and class in C#?
Structs vs classes in C#
Structs may seem similar to classes, but there are important differences that you should be aware of. First of all, classes are reference types and structs are value types. By using structs, you can create objects that behave like the built-in types and enjoy their benefits as well.
- When you call the New operator on a class, it will be allocated on the heap. However, when you instantiate a struct, it gets created on the stack. This will yield performance gains. Also, you will not be dealing with references to an instance of a struct as you would with classes. You will be working directly with the struct instance. Because of this, when passing a struct to a method, it's passed by value instead of as a reference.
- Structs can declare constructors, but they must take parameters. It is an error to declare a default (parameterless) constructor for a struct. Struct members cannot have initializers. A default constructor is always provided to initialize the struct members to their default values.
- When you create a struct object using the New operator, it gets created and the appropriate constructor is called. Unlike classes, structs can be instantiated without using the New operator. If you do not use New, the fields will remain unassigned and the object cannot be used until all the fields are initialized.
- There is no inheritance for structs as there is for classes. A struct cannot inherit from another struct or class, and it cannot be the base of a class. Structs, however, inherit from the base class object. A struct can implement interfaces, and it does that exactly as classes do,
- Structs are simple to use and can prove to be useful at times. Just keep in mind that they're created on the stack and that you're not dealing with references to them but dealing directly with them. Whenever you have a need for a type that will be used often and is mostly just a piece of data, structs might be a good option.
How do I call one constructor from another in C#?
You use : base (parameters) or : this (parameters) just before the actual code for the constructor, depending on whether you want to call a constructor in the base class or in this class.
What is the difference between indexers and properties in C#?
Comparison Between Properties and Indexers
Indexers are similar to properties. Except for the differences shown in the following , all of the rules defined for property accessors apply to indexer accessors as well.
Properties | Indexers |
Identified by its name. | Identified by its signature. |
Accessed through a simple name or a member access. | Accessed through an element access. |
Can be a static or an instance member. | Must be an instance member. |
A get accessor of a property has no parameters. | A get accessor of an indexer has the same formal parameter list as the indexer. |
A set accessor of a property contains the implicit value parameter. | A set accessor of an indexer has the same formal parameter list as the indexer, in addition to the value parameter. |
Which interface(s) must a class implement in order to support the foreach statement?
Required interface for foreach statement:
A class must implement the IEnumerable and IEnumeratorinterfaces to support the foreach statement.
Explain Abstract, Sealed, and Static Modifiers in C#.
C# provides many modifiers for use with types and type members. Of these, three can be used with classes: abstract, sealed and static.
abstract
Indicates that a class is to be used only as a base class for other classes. This means that you cannot create an instance of the class directly. Any class derived from it must implement all of its abstract methods and accessors. Despite its name, an abstract class can possess non-abstract methods and properties.
sealed
Specifies that a class cannot be inherited (used as a base class). Note that .NET does not permit a class to be both abstract and sealed.
static
Specifies that a class contains only static members (.NET 2.0).
What's the difference between override and new in C#?
This is all to do with polymorphism. When a virtual method is called on a reference, the actual type of the object that the reference refers to is used to decide which method implementation to use. When a method of a base class is overridden in a derived class, the version in the derived class is used, even if the calling code didn't "know" that the object was an instance of the derived class. For instance:
{
public virtual void SomeMethod()
{
}
}
public class Derived : Base
{
public override void SomeMethod()
{
}
}
...
Base b = new Derived();
b.SomeMethod();
will end up calling Derived.SomeMethod if that overrides Base.SomeMethod.
Now, if you use the new keyword instead of override, the method in the derived class doesn't override the method in the base class, it merely hides it. In that case, code like this:
public class Base
{
public virtual void SomeOtherMethod()
{
}
}
public class Derived : Base
{
public new void SomeOtherMethod()
{
}
}
...
Base b = new Derived();
Derived d = new Derived();
b.SomeOtherMethod();
d.SomeOtherMethod();
Will first call Base.SomeOtherMethod , then Derived.SomeOtherMethod . They're effectively two entirely separate methods which happen to have the same name, rather than the derived method overriding the base method.
If you don't specify either new or overrides, the resulting output is the same as if you specified new, but you'll also get a compiler warning (as you may not be aware that you're hiding a method in the base class method, or indeed you may have wanted to override it, and merely forgot to include the keyword)
Name two ways that you can prevent a class from being instantiated.
Ways to prevent a class from instantiated:
A class cannot be instantiated if it is abstract or if it has a private constructor.
Explain some features of interface in C#.
Comparison of interface with class (interface vs class):
- An interface cannot inherit from a class.
- An interface can inherit from multiple interfaces.
- A class can inherit from multiple interfaces, but only one class.
- Interface members must be methods, properties, events, or indexers.
- All interface members must have public access (the default).
- By convention, an interface name should begin with an uppercase I.
Can an abstract class have non-abstract methods?
An abstract class may contain both abstract and non-abstractmethods. But an interface can contain only abstract methods.
How do I use an alias for a namespace or class in C#?
Use the using directive to create an alias for a long namespace or class. You can then use it anywhere you normally would have used that class or namespace. The using alias has a scope within the namespace you declare it in.
Sample code:
// Namespace:
using act = System.Runtime.Remoting.Activation;
// Class:
using list = System.Collections.ArrayList;
...
list l = new list(); // Creates an ArrayList
act.UrlAttribute obj; // Equivalent to System.Runtime.Remoting.Activation.UrlAttribute obj
What type of class cannot be inherited?
A sealed class cannot be inherited. A sealed class is used primarily when the class contains static members. Note that a struct is implicitly sealed; so they cannot be inherited.
What keyword must a derived class use to replace a non-virtual inherited method?
new keyword is used to replace (not override) an inherited method with one of the same name.
Object Oriented Programming Question Usually asked in Interview
There are two types of polymorphism one is compile time polymorphism and the other is run time polymorphism. Compile time polymorphism is functions and operators overloading. Runtime time polymorphism is done using inheritance and virtual functions. Here are some ways how we implement polymorphism in Object Oriented programming languages
Interface and abstract methods
Like in C# or JAVA different classes implement a common interface in different ways; is an example of Runtime polymorphism. The interface defines the abstract member functions (no implementation). In class that implement the interface; we define body of those abstract members according to the requirement. Means single definition in interface but multiple implementation in child classes.
Virtual member functions
Using virtual member functions in an inheritance hierarchy allows run-time selection of the appropriate member function. A virtual function is a member function of the base class and which is redefined by the derived class. Such functions can have different implementations that are invoked by a run-time determination of the subtype (virtual method invocation, dynamic binding).
A virtual function has a different functionality in the derived class according to the requirement. The virtual function within the base class provides the form of the interface to the function. Virtual function implements the philosophy of one interface and multiple methods (polymorphism).
The virtual functions are resolved at the run time. This is called dynamic binding. The functions which are not virtual are resolved at compile time which is called static binding. A virtual function is created using the keyword virtual which precedes the name of the function.
A process, encapsulation means the act of enclosing one or more items within a (physical or logical) container (Class).
Object-oriented programming is based on encapsulation. When an objects state and behavior are kept together, they are encapsulated. That is, the data that represents the state of the object and the methods (Functions and Subs) that manipulate that data are stored together as a cohesive unit.
The object takes requests from other client objects, but does not expose its the details of its data or code to them. The object alone is responsible for its own state, exposing public messages for clients, and declaring private methods that make up its implementation. The client depends on the (hopefully) simple public interface, and does not know about or depend on the details of the implementation.
For example, a HashTable object will take get() and set() requests from other objects, but does not expose its internal hash table data structures or the code strategies that it uses.
Encapsulation makes it possible to separate an objects implementation from its behavior to restrict access to its internal data. This restriction allows certain details of an objects behavior to be hidden. It allows us to create a "black box" and protects an objects internal state from corruption by its clients.
Encapsulation is a technique for minimizing interdependencies among modules by defining a strict external interface. This way, internal coding can be changed without affecting the interface, so long as the new implementation supports the same (or upwards compatible) external interface. So encapsulation prevents a program from becoming so interdependent that a small change has massive ripple effects.
The implementation of an object can be changed without affecting the application that uses it for: Improving performance, fix a bug, consolidate code or for porting.
You're not allowed any fields in an interface, not even static ones. A field is an implementation of an object attribute.
You're not allowed any constructors in an interface. A constructor contains the statements used to initialize the fields in an object, and an interface does not contain any fields!
You're not allowed a destructor in an interface. A destructor contains the statements used to destroy an object instance.
You cannot supply an access modifier. All methods in an interface are implicitly public.
You cannot nest any types (enums, structs, classes, interfaces, or delegates) inside an interface.
You're not allowed to inherit an interface from a struct or a class. Structs and classes contain implementation; if an interface were allowed to inherit from either, it would be inheriting some implementation
An object can be considered a "thing" that can perform a set of activities. The set of activities that the object performs defines the object's behavior.
The state of the object changes according to the methods which are applied to it. We refer to these possible sequences of state changes as the behavior of the object. So the behavior of an object is defined by the set of methods which can be applied on it.
Objects can communicate by passing messages to each other.
If class A inherits from class B, then B is called superclass of A. A is called subclass of B. Objects of a subclass can be used where objects of the corresponding superclass are expected. This is due to the fact that objects of the subclass share the same behavior as objects of the superclass.
However, subclasses are not limited to the state and behaviors provided to them by their superclass. Subclasses can add variables and methods to the ones they inherit from the superclass.
In the literature you may also find other terms for "superclass" and "subclass". Superclasses are also called parent classes or base classes. Subclasses may also be called child classes or just derived classes.
Inheritance Example
Like a car, truck or motorcycles have certain common characteristics- they all have wheels, engines and brakes. Hence they all could be represented by a common class Vehicle which encompasses all those attributes and methods that are common to all types of vehicles.
However they each have their own unique attributes; car has 4 wheels and is smaller is size to a truck; whereas a motorcycle has 2 wheels. Thus we see a parent-child type of relationship here where the Car, Truck or Motorcycle can inherit certain Characteristics from the parent Vehicle; at the same time having their own unique attributes. This forms the basis of inheritance; Vehicle is the Parent, Super or the Base class. Car, Truck and Motorcycle become the Child, Sub or the Derived class.
Some important aspects of inheritance are:
Inheritance is transitive. If C is derived from B, and B is derived from A, then C inherits the members declared in B as well as the members declared in A.
A derived class extends its direct base class. A derived class can add new members to those it inherits, but it cannot remove the definition of an inherited member.
Constructors and destructors are not inherited, but all other members are, regardless of their declared accessibility. However, depending on their declared accessibility, inherited members might not be accessible in a derived class.
A derived class can hide inherited members by declaring new members with the same name or signature. Note however that hiding an inherited member does not remove that member; it merely makes that member inaccessible in the derived class.
An instance of a class contains a set of all instance fields declared in the class and its base classes, and an implicit conversion exists from a derived class type to any of its base class types. Thus, a reference to an instance of some derived class can be treated as a reference to an instance of any of its base classes.
A class can declare virtual methods, properties, and indexers, and derived classes can override the implementation of these function members. This enables classes to exhibit polymorphic behavior wherein the actions performed by a function member invocation varies depending on the run-time type of the instance through which that function member is invoked.
Implementing an interface allows a class to become more formal about the behavior it promises to provide. Interfaces form a contract between the class and the outside world, and this contract is enforced at build time by the compiler. If your class claims to implement an interface, all methods defined by that interface must appear in its source code before the class will successfully compile.
We can not make instance of Abstract Class as well as Interface. They only allow other classes to inherit from them. And abstract functions must be overridden by the implemented classes. Here are some differences in abstract class and interface.
An interface cannot provide code of any method or property, just the signature. We dont need to put abstract and public keyword. All the methods and properties defined in Interface are by default public and abstract. An abstract class can provide complete code of methods but there must exist a method or property without body.
A class can implement several interfaces but can inherit only one abstract class. Means multiple inheritance is possible in .Net through Interfaces.
Here are some more features of static class:
• Static classes only contain static members.
• Static classes can not be instantiated. They cannot contain Instance Constructors
• Static classes are sealed.
To access a static class member, use the name of the class instead of an instance variable
Static methods and Static properties can only access static fields and static events.
Like: int i = Car.GetWheels;
Here Car is class name and GetWheels is static property.
Static members are often used to represent data or calculations that do not change in response to object state.
A reference parameter is used for "by reference" parameter passing, in which the parameter acts as an alias for a caller-provided argument. A reference parameter does not itself define a variable, but rather refers to the variable of the corresponding argument. Modifications of a reference parameter impact the corresponding argument.
A parameter array is declared with a params modifier in C#. There can be only one parameter array for a given method, and it must always be the last parameter specified. The type of a parameter array is always a single dimensional array type. A caller can either pass a single argument of this array type, or any number of arguments of the element type of this array type.
In C# a reference parameter is declared with a ref modifier but an output parameter is declared with an out modifier.
Constants are permitted to depend on other constants within the same program as long as there are no circular dependencies. The example
class Constants
{
public const int A = 1;
public const int B = A + 1;
}
shows a class named Constants that has two public constants
const vs. readonly
const and readonly perform a similar function on data members, but they have a few important differences.
const
A constant member is defined at compile time and cannot be changed at runtime. Constants are declared as a field, using the const keyword and must be initialized as they are declared. For example;
public class MyClass
{
public const double PI = 3.14159;
}
PI cannot be changed in the application anywhere else in the code as this will cause a compiler error.
Constants must be of an integral type (sbyte, byte, short, ushort, int, uint, long, ulong, char, float, double, decimal,bool, or string), an enumeration, or a reference to null.
Since classes or structures are initialized at run time with the new keyword, and not at compile time, you can't set a constant to a class or structure.
Constants can be marked as public, private, protected, internal, or protected internal.
Constants are accessed as if they were static fields, although they cannot use the static keyword.
To use a constant outside of the class that it is declared in, you must fully qualify it using the class name.
readonly
A read only member is like a constant in that it represents an unchanging value. The difference is that a readonly member can be initialized at runtime, in a constructor as well being able to be initialized as they are declared. For example:
public class MyClass
{
public readonly double PI = 3.14159;
}
or
public class MyClass
{
public readonly double PI;
public MyClass()
{
PI = 3.14159;
}
}
Because a readonly field can be initialized either at the declaration or in a constructor, readonly fields can have different values depending on the constructor used. A readonly field can also be used for runtime constants as in the following example:
public static readonly uint l1 = (uint)DateTime.Now.Ticks;
Notes
readonly members are not implicitly static, and therefore the static keyword can be applied to a readonly field explicitly if required.
A readonly member can hold a complex object by using the new keyword at initialization.
readonly members cannot hold enumerations.
static
Use of the static modifier to declare a static member, means that the member is no longer tied to a specific object. This means that the member can be accessed without creating an instance of the class. Only one copy of static fields and events exists, and static methods and properties can only accessstatic fields and static events. For example:
public class Car
{
public static int NumberOfWheels = 4;
}
The static modifier can be used with classes, fields, methods, properties, operators, events and constructors, but cannot be used with indexers, destructors, or types other than classes.
static members are initialized before the static member is accessed for the first time, and before the static constructor, if any is called. To access a staticclass member, use the name of the class instead of a variable name to specify the location of the member. For example:
int i = Car.NumberOfWheels;
Methods can be overloaded, which means that multiple methods may have the same name so long as they have unique signatures. The signature of a method consists of the name of the method and the number, modifiers, and types of its formal parameters. The signature of a method does not include the return type.
Properties are a natural extension of fields. Both are named members with associated types, and the syntax for accessing fields and properties is the same. However, unlike fields, properties do not denote storage locations. Instead, properties have accessors that specify the statements to be executed when their values are read or written.
Properties are defined with property declarations. The first part of a property declaration looks quite similar to a field declaration. The second part includes a get accessor and/or a set accessor.
Properties that can be both read and written, include both get and set accessors. The get accessor is called when the property's value is read; the set accessor is called when the property's value is written. In a set accessor, the new value for the property is made available via an implicit parameter named value.